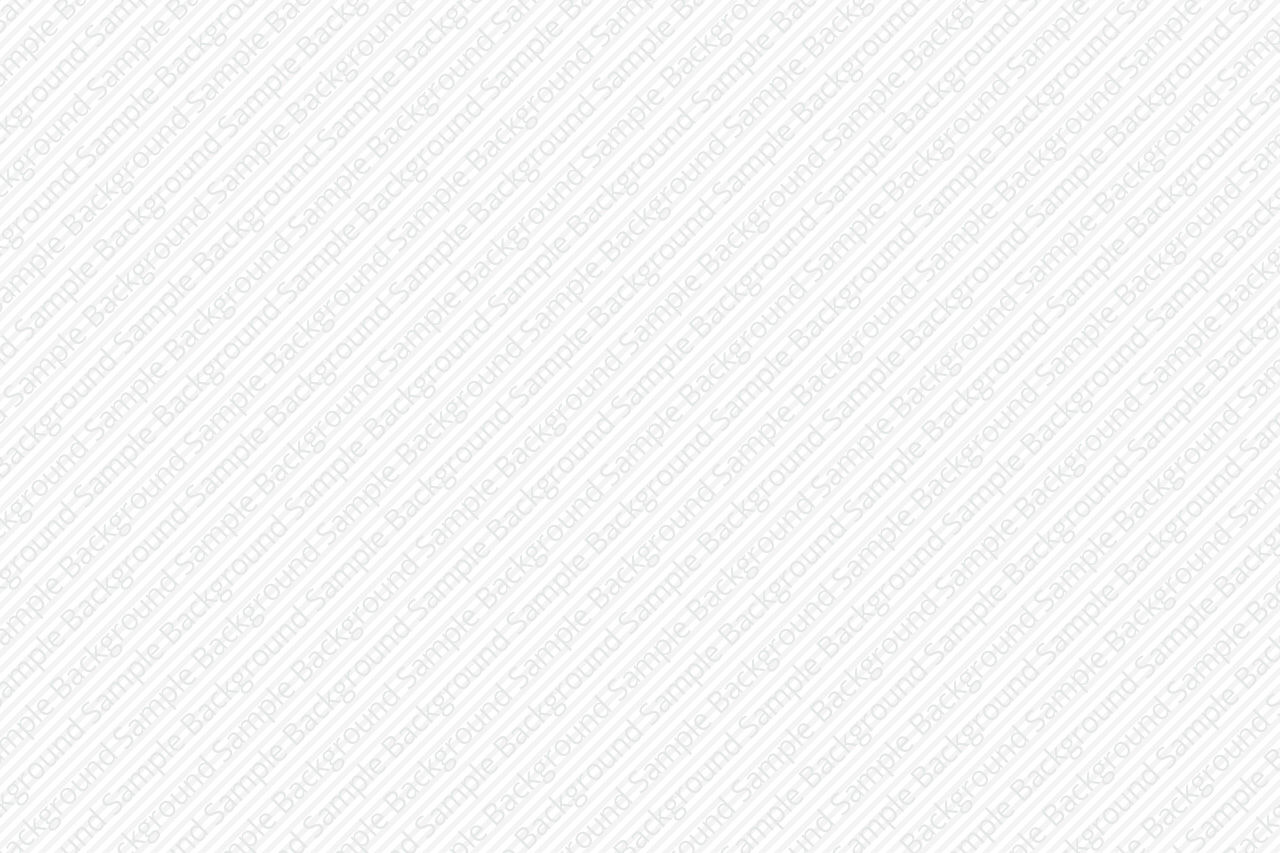
Практикум по программированию на языке С,
Вычислительная физика, Теория алгоритмов.
ФНБИК МФТИ
2015-2016 учебный год
1 #include<stdio.h>
2 #include<ctype.h>
3 #include<string.h>
4 #include<stdlib.h>
5
6 #define MAXLINE 80
7
8 int readline(char *);
9 int my_atoi(char *);
10
11 int main(void){
12 char *error="Can not allocate memory";
13 char yli[]="Your line is";
14 char *l;
15
16 char *line;
17
18 if( (line=(char *) malloc(MAXLINE*sizeof(char)) ) == NULL){
19 /* if( ! (line= (char *) malloc(MAXLINE*sizeof(char)) ) );
20 has the same meaning */
21 printf("%s",error);
22 return -1;
23 }
24
25 if(readline(line)){
26
27 printf("%s %s its length is %d \n",yli,line,strlen(line));
28 printf("the characters in the string are :\n");
29
30 for(l=line;*l!=0 ;l++)
31 printf("char %c, dec %d, oct %o, hex %x\n",*l,*l,*l,*l);
32 printf("Your integer number is %d\n",my_atoi(line));
33 }
34 return 0;
35 }
36
37 int readline(char *line){
38 int ijk=0;
39 char c;
40
41 c=getchar();
42 while(isspace(c))
43 c=getchar();
44
45 while(isdigit ( c )){
46 *line=c;
47 line++;
48 ijk++;
49
50 if(ijk==MAXLINE){
51 printf("The maximum length of line exceeded\n");
52 return 0;
53 }
54
55 c=getchar();
56 }
57 *line='\0'; /* simply *line=0 might be here */
58 return ijk;
59 }
60
61 int my_atoi(char *line){
62
63 int c,n,result=0;
64 while((c=*line++)!='\0'){
65
66 switch(c){
67 case '0' : n=0; break;
68 case '1' : n=1; break;
69 case '2' : n=2; break;
70 case '3' : n=3; break;
71 case '4' : n=4; break;
72 case '5' : n=5; break;
73 case '6' : n=6; break;
74 case '7' : n=7; break;
75 case '8' : n=8; break;
76 case '9' : n=9; break;
77 default : ;
78 }
79
80 result=result*10+n;
81 }
82 return result;
83 }
84
85 //======================================================================
86 /*
87 1 int my_atoi(char *line){
88 2
89 3 int c,result=0;
90 4 while((c=*line++)!='\0')
91 5 result=result*10+(c-'0');
92 6
93 7 return result;
94 8 }
95 */